Cg 编程/Unity/球体的软阴影

本教程涵盖球体的软阴影。
这是关于光照的几个教程之一,这些教程超出了 Phong 反射模型,Phong 反射模型是一种局部光照模型,因此没有考虑阴影。所提出的技术渲染单个球体在任何网格上的软阴影,并且与 Orion Sky Lawlor 提出的技术有一定的关系(参见“进一步阅读”部分)。该着色器可以扩展以渲染少量球体的阴影,但这会以渲染性能为代价;然而,它不能轻松地应用于任何其他类型的阴影投射器。潜在的应用包括电脑球类游戏(球通常是唯一需要软阴影的物体,也是唯一应该在所有其他物体上投射动态阴影的物体),具有球形主角的电脑游戏(例如,“弹球狂热”),仅由球体组成的可视化效果(例如,行星可视化、小原子核、原子或分子的球体模型等),或者可以填充球体并受益于软阴影的测试场景。
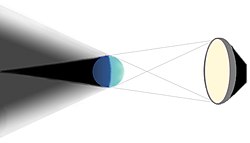

虽然定向光源和点光源会产生硬阴影,但任何面积光源都会产生软阴影。对于所有真实的光源,特别是太阳和任何灯泡或灯,情况也是如此。在阴影投射器后面的某些点,光源的任何部分都不可见,阴影均匀地变暗:这是本影。从其他点,光源或多或少可见,因此阴影或多或少完整:这是半影。最后,有些点可以看见光源的整个面积:这些点位于阴影之外。
在许多情况下,阴影的柔和度主要取决于阴影投射器和阴影接收器之间的距离:距离越大,阴影越柔和。这是艺术中众所周知的现象;例如,参见卡拉瓦乔的右侧绘画。

我们将近似计算表面上一个点的阴影,当半径为 的球体在S(相对于表面点)处遮挡半径为 的球形光源在L(相对于表面点)处时;参见左侧的图形。
为此,我们考虑一个方向为T、经过表面点的球体切线。此外,选择此切线位于L和S所跨越的平面上,即平行于左侧图形的视图平面。关键的观察结果是光源中心的最小距离 和这条切线直接与表面点的阴影量相关,因为它决定了从表面点可见的光源区域的大小。更确切地说,我们需要一个带符号的距离(如果切线在与球体相同的一侧的L上,则为正,否则为负)来确定表面点是在本影中 (),半影中 (),还是阴影之外 ()。
为了计算 ,我们考虑L和S之间的角度以及T和S之间的角度。这两个角度之差是L和T之间的角度,它与 的关系为
.
因此,到目前为止,我们有
我们可以使用以下公式计算 **T** 和 **S** 之间的角度
.
因此
.
对于 **L** 和 **S** 之间的角度,我们使用向量叉积的一个性质
.
因此
.
总而言之,我们有
我们到目前为止所做的近似并不重要;更重要的是,它不会产生渲染伪影。 如果性能是一个问题,我们可以更进一步地使用 arcsin(x) ≈ x;即,我们可以使用
这避免了所有三角函数;但是,它确实引入了渲染伪影(特别是在面向光源的半影中存在镜面高光时)。 这些渲染伪影是否值得性能提升,需要在每种情况下进行判断。
接下来,我们看看如何根据 计算阴影程度 。当 从 减小到 时, 应该从 0 增加到 1。换句话说,我们希望在 的 -1 到 1 之间的值之间实现一个平滑的过渡。可能是实现这一点最有效的方法是使用内置 Cg 函数 smoothstep(a,b,x) = t*t*(3-2*t)
提供的 Hermite 插值,其中 t=clamp((x-a)/(b-a),0,1)
虽然这不是 和 之间基于物理的关联的特别好的近似,但它仍然能很好地体现基本特征。
此外,如果光线方向 L 与 S 的方向相反,即它们的点积为负,则 应该为 0。这个条件实际上有点棘手,因为它会导致 L 和 S 正交的平面上出现明显的间断。为了平滑这种间断,我们可以再次使用 smoothstep
来计算一个改进的值
此外,如果点光源距离表面点比遮挡球体更近,则我们必须将 设置为 0。这同样有点棘手,因为球形光源可能会与投射阴影的球体相交。一个避免过于明显的伪影(但无法处理完全相交问题)的解决方案是
对于方向光源,我们只需设置 。然后, 表示无阴影照明水平,应该乘以光源的任何照明。(因此,环境光不应该乘以这个因子。)如果计算多个阴影投射者的阴影,则需要将所有阴影投射者的 项组合起来,用于每个光源。通常的方法是将它们相乘,虽然这可能不准确(尤其是在阴影重叠时)。
实现计算 lightDirection
和 sphereDirection
向量的长度,然后使用归一化向量进行处理。这样,这些向量的长度只需要计算一次,我们甚至可以避免一些除法运算,因为我们可以使用归一化向量。以下是片段着色器的关键部分
// computation of level of shadowing w
float3 sphereDirection =
_SpherePosition.xyz - input.posWorld.xyz;
float sphereDistance = length(sphereDirection);
sphereDirection = sphereDirection / sphereDistance;
float d = lightDistance
* (asin(min(1.0,
length(cross(lightDirection, sphereDirection))))
- asin(min(1.0, _SphereRadius / sphereDistance)));
float w = smoothstep(-1.0, 1.0, -d / _LightSourceRadius);
w = w * smoothstep(0.0, 0.2,
dot(lightDirection, sphereDirection));
if (0.0 != _WorldSpaceLightPos0.w) // point light source?
{
w = w * smoothstep(0.0, _SphereRadius,
lightDistance - sphereDistance);
}
使用 asin(min(1.0, ...))
确保 asin
的参数在允许的范围内。
完整的源代码定义了阴影投射球体和光源半径的属性。所有值都应以世界坐标表示。对于方向光源,光源半径应以弧度表示(1 rad = 180° / π)。设置阴影投射球体的位置和半径的最佳方法是简短的脚本,该脚本应附加到使用该着色器的所有接收阴影的对象,例如
@script ExecuteInEditMode()
var occluder : GameObject;
function Update () {
if (null != occluder) {
GetComponent(Renderer).sharedMaterial.SetVector("_SpherePosition",
occluder.transform.position);
GetComponent(Renderer).sharedMaterial.SetFloat("_SphereRadius",
occluder.transform.localScale.x / 2.0);
}
}
该脚本具有一个公共变量 occluder
,该变量应设置为阴影投射球体。然后,它设置以下着色器的属性 _SpherePostion
和 _SphereRadius
(该着色器应附加到与脚本相同的接收阴影对象)。
片段着色器相当长,实际上我们必须使用 #pragma target 3.0
行来忽略旧版 GPU 的一些限制,如 Unity 参考 中所述。
Shader "Cg shadow of sphere" {
Properties {
_Color ("Diffuse Material Color", Color) = (1,1,1,1)
_SpecColor ("Specular Material Color", Color) = (1,1,1,1)
_Shininess ("Shininess", Float) = 10
_SpherePosition ("Sphere Position", Vector) = (0,0,0,1)
_SphereRadius ("Sphere Radius", Float) = 1
_LightSourceRadius ("Light Source Radius", Float) = 0.005
}
SubShader {
Pass {
Tags { "LightMode" = "ForwardBase" }
// pass for ambient light and first light source
CGPROGRAM
#pragma vertex vert
#pragma fragment frag
#pragma target 3.0
#include "UnityCG.cginc"
uniform float4 _LightColor0;
// color of light source (from "Lighting.cginc")
// User-specified properties
uniform float4 _Color;
uniform float4 _SpecColor;
uniform float _Shininess;
uniform float4 _SpherePosition;
// center of shadow-casting sphere in world coordinates
uniform float _SphereRadius;
// radius of shadow-casting sphere
uniform float _LightSourceRadius;
// in radians for directional light sources
struct vertexInput {
float4 vertex : POSITION;
float3 normal : NORMAL;
};
struct vertexOutput {
float4 pos : SV_POSITION;
float4 posWorld : TEXCOORD0;
float3 normalDir : TEXCOORD1;
};
vertexOutput vert(vertexInput input)
{
vertexOutput output;
float4x4 modelMatrix = unity_ObjectToWorld;
float4x4 modelMatrixInverse = unity_WorldToObject;
output.posWorld = mul(modelMatrix, input.vertex);
output.normalDir = normalize(
mul(float4(input.normal, 0.0), modelMatrixInverse).xyz);
output.pos = mul(UNITY_MATRIX_MVP, input.vertex);
return output;
}
float4 frag(vertexOutput input) : COLOR
{
float3 normalDirection = normalize(input.normalDir);
float3 viewDirection = normalize(
_WorldSpaceCameraPos - input.posWorld.xyz);
float3 lightDirection;
float lightDistance;
float attenuation;
if (0.0 == _WorldSpaceLightPos0.w) // directional light?
{
attenuation = 1.0; // no attenuation
lightDirection =
normalize(_WorldSpaceLightPos0.xyz);
lightDistance = 1.0;
}
else // point or spot light
{
lightDirection =
_WorldSpaceLightPos0.xyz - input.posWorld.xyz;
lightDistance = length(lightDirection);
attenuation = 1.0 / lightDistance; // linear attenuation
lightDirection = lightDirection / lightDistance;
}
// computation of level of shadowing w
float3 sphereDirection =
_SpherePosition.xyz - input.posWorld.xyz;
float sphereDistance = length(sphereDirection);
sphereDirection = sphereDirection / sphereDistance;
float d = lightDistance
* (asin(min(1.0,
length(cross(lightDirection, sphereDirection))))
- asin(min(1.0, _SphereRadius / sphereDistance)));
float w = smoothstep(-1.0, 1.0, -d / _LightSourceRadius);
w = w * smoothstep(0.0, 0.2,
dot(lightDirection, sphereDirection));
if (0.0 != _WorldSpaceLightPos0.w) // point light source?
{
w = w * smoothstep(0.0, _SphereRadius,
lightDistance - sphereDistance);
}
float3 ambientLighting =
UNITY_LIGHTMODEL_AMBIENT.rgb * _Color.rgb;
float3 diffuseReflection =
attenuation * _LightColor0.rgb * _Color.rgb
* max(0.0, dot(normalDirection, lightDirection));
float3 specularReflection;
if (dot(normalDirection, lightDirection) < 0.0)
// light source on the wrong side?
{
specularReflection = float3(0.0, 0.0, 0.0);
// no specular reflection
}
else // light source on the right side
{
specularReflection = attenuation * _LightColor0.rgb
* _SpecColor.rgb * pow(max(0.0, dot(
reflect(-lightDirection, normalDirection),
viewDirection)), _Shininess);
}
return float4(ambientLighting
+ (1.0 - w) * (diffuseReflection + specularReflection),
1.0);
}
ENDCG
}
Pass {
Tags { "LightMode" = "ForwardAdd" }
// pass for additional light sources
Blend One One // additive blending
CGPROGRAM
#pragma vertex vert
#pragma fragment frag
#pragma target 3.0
#include "UnityCG.cginc"
uniform float4 _LightColor0;
// color of light source (from "Lighting.cginc")
// User-specified properties
uniform float4 _Color;
uniform float4 _SpecColor;
uniform float _Shininess;
uniform float4 _SpherePosition;
// center of shadow-casting sphere in world coordinates
uniform float _SphereRadius;
// radius of shadow-casting sphere
uniform float _LightSourceRadius;
// in radians for directional light sources
struct vertexInput {
float4 vertex : POSITION;
float3 normal : NORMAL;
};
struct vertexOutput {
float4 pos : SV_POSITION;
float4 posWorld : TEXCOORD0;
float3 normalDir : TEXCOORD1;
};
vertexOutput vert(vertexInput input)
{
vertexOutput output;
float4x4 modelMatrix = unity_ObjectToWorld;
float4x4 modelMatrixInverse = unity_WorldToObject;
output.posWorld = mul(modelMatrix, input.vertex);
output.normalDir = normalize(
mul(float4(input.normal, 0.0), modelMatrixInverse).xyz);
output.pos = mul(UNITY_MATRIX_MVP, input.vertex);
return output;
}
float4 frag(vertexOutput input) : COLOR
{
float3 normalDirection = normalize(input.normalDir);
float3 viewDirection = normalize(
_WorldSpaceCameraPos - input.posWorld.xyz);
float3 lightDirection;
float lightDistance;
float attenuation;
if (0.0 == _WorldSpaceLightPos0.w) // directional light?
{
attenuation = 1.0; // no attenuation
lightDirection = normalize(_WorldSpaceLightPos0.xyz);
lightDistance = 1.0;
}
else // point or spot light
{
lightDirection =
_WorldSpaceLightPos0.xyz - input.posWorld.xyz;
lightDistance = length(lightDirection);
attenuation = 1.0 / lightDistance; // linear attenuation
lightDirection = lightDirection / lightDistance;
}
// computation of level of shadowing w
float3 sphereDirection =
_SpherePosition.xyz - input.posWorld.xyz;
float sphereDistance = length(sphereDirection);
sphereDirection = sphereDirection / sphereDistance;
float d = lightDistance
* (asin(min(1.0,
length(cross(lightDirection, sphereDirection))))
- asin(min(1.0, _SphereRadius / sphereDistance)));
float w = smoothstep(-1.0, 1.0, -d / _LightSourceRadius);
w = w * smoothstep(0.0, 0.2,
dot(lightDirection, sphereDirection));
if (0.0 != _WorldSpaceLightPos0.w) // point light source?
{
w = w * smoothstep(0.0, _SphereRadius,
lightDistance - sphereDistance);
}
float3 diffuseReflection =
attenuation * _LightColor0.rgb * _Color.rgb
* max(0.0, dot(normalDirection, lightDirection));
float3 specularReflection;
if (dot(normalDirection, lightDirection) < 0.0)
// light source on the wrong side?
{
specularReflection = float3(0.0, 0.0, 0.0);
// no specular reflection
}
else // light source on the right side
{
specularReflection = attenuation * _LightColor0.rgb
* _SpecColor.rgb * pow(max(0.0, dot(
reflect(-lightDirection, normalDirection),
viewDirection)), _Shininess);
}
return float4((1.0 - w) * (diffuseReflection
+ specularReflection), 1.0);
}
ENDCG
}
}
Fallback "Specular"
}
恭喜!我希望你成功渲染了一些漂亮的柔和阴影。我们已经了解了
- 什么是柔和阴影,以及什么是半影和本影。
- 如何计算球体的柔和阴影。
- 如何实现计算,包括使用 JavaScript 编写的脚本,该脚本根据另一个
GameObject
设置一些属性。
如果你还想了解更多
- 关于着色器代码的其余部分,你应该阅读 部分“平滑镜面高光”.
- 关于柔和阴影的计算,你应该阅读 Orion Sky Lawlor 的出版物:“插值友好的柔和阴影贴图”,发表在计算机图形和虚拟现实 ’06 会议论文集,第 111–117 页。可以在 网上 找到预印本。